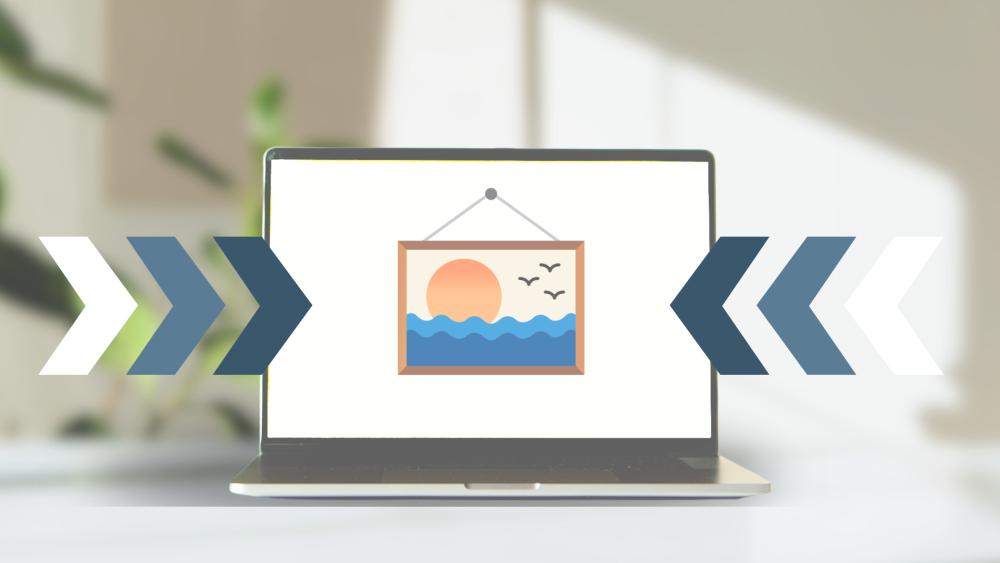
There is a number of things that even the most experienced developers have to look up all the time. One of these things is to center elements in CSS. This post is summarizing the solutions to how you can center your HTML elements on your website, so you have a place you can come back to to look them up.
You can watch the content as a YouTube Video here:
How to Center Text in a Div
So you have a <div>
and inside that div you have some text. How can you center this text?
<div class="centered">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Dolorem minus alias repudiandae velit nobis, itaque, quam ratione facere rerum veniam nisi excepturi maiores suscipit obcaecati aperiam beatae voluptate molestias vero.
</div>
The simplest solution to this is: by using text-align: center
. Like so:
.centered {
text-align: center;
}
This will result in neatly centered text:
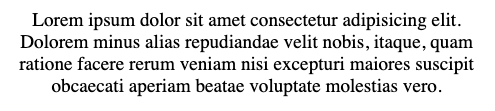
This also works for the case where your text is in a <p>
element:
<div class="centered">
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Dolorem minus alias repudiandae velit nobis, itaque, quam ratione facere rerum veniam nisi excepturi maiores suscipit obcaecati aperiam beatae voluptate molestias vero.</p>
</div>
And it also works if you have many nested <div>
s, like so:
<div class="centered">
<div>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Dolorem minus alias repudiandae velit nobis, itaque, quam ratione facere rerum veniam nisi excepturi maiores suscipit obcaecati aperiam beatae voluptate molestias vero.</p>
</div>
</div>
Same result:
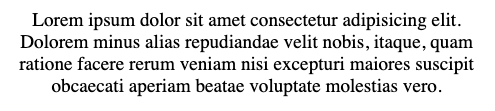
This is because the text-align: center
style is inherited by all nested child elements.
So centering text is easy. But how about images?
How to Center an Image in a Div
You could, of course, center an <img>
by putting text-align: center
on its parent <div>
as well. But then, everything in that parent div
becomes centered, like this:
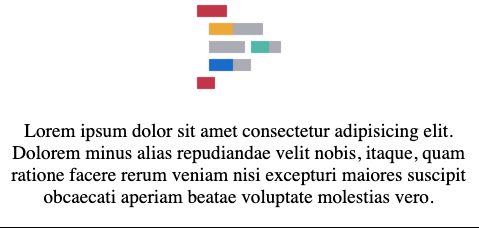
If you only want to center your <img>
and not the other stuff, you need a different approach.
<div class="container">
<img src="images/logo.png" alt="HCS Logo" class="centered-image">
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Incidunt doloremque, voluptates maxime a dolorem eum dignissimos consequuntur quas amet modi atque, accusantium pariatur, id velit tempore quasi explicabo ab suscipit!</p>
</div>
In the CSS, you do change the style of the image to this:
.centered-image {
display: block;
margin-left: auto;
margin-right: auto;
}
This centers your image while leaving your text left-aligned.
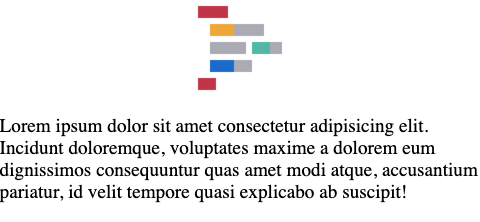
Perfect, right? 😄
How to Center a Div in a Div?
The next problem we might face is if we have a <div>
and we want to center it inside a <div>
.
<div class="parent">
<div class="child">
Lorem ipsum dolor sit amet consectetur, adipisicing elit. Aut eveniet veritatis quibusdam illum autem ab officiis rerum obcaecati placeat sit. Temporibus non vitae inventore dolore tempore saepe natus voluptatem velit.
</div>
</div>
Let’s illustrate this by using different background colors for each div
and put a little bit of padding inside the div
s, so we can see better what’s going on.
.parent {
background-color: aquamarine;
padding: 1rem;
}
.child {
background-color: cadetblue;
padding: 1rem;
width: 200px;
}
I also set a width
to the child element, so you can see the effect better.
This will look like this:
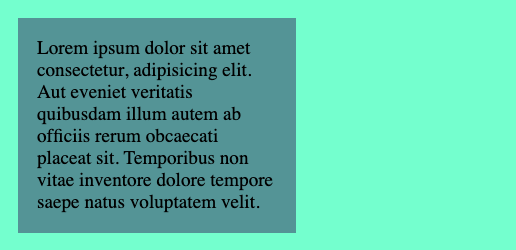
Now, we can apply the same trick with the margin to center the child div
:
.parent {
background-color: aquamarine;
padding: 1rem;
}
.child {
background-color: cadetblue;
margin-left: auto;
margin-right: auto;
padding: 1rem;
width: 200px;
}
And as a result we get a centered div
inside a div
!
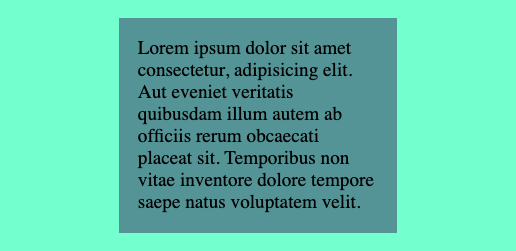
How to Center Things with Flexbox?
If you are using Flexbox in your layout anyway, a better solution might be to center your elements using Flexbox.
In Flexbox, you have a parent container and a child element, like so:
<div class="container">
<div class="element">
Lorem ipsum dolor sit amet consectetur, adipisicing elit. Aut eveniet veritatis quibusdam illum autem ab officiis rerum obcaecati placeat sit. Temporibus non vitae inventore dolore tempore saepe natus voluptatem velit.
</div>
</div>
.container {
display: flex;
background-color: aquamarine;
padding: 1rem;
}
.element {
background-color: cadetblue;
padding: 1rem;
width: 200px;
}
We put a smaller size on the .element
, just so that we can see the effect of centering better.
This results in this layout:
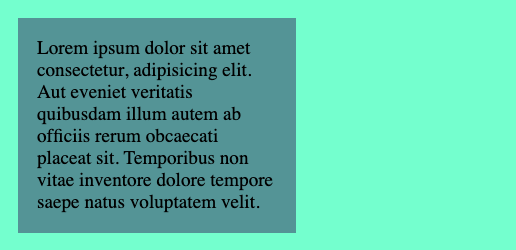
Now, to center the child element, we can use Flexbox’ justify-content
property on the parent container. Like so:
.container {
display: flex;
justify-content: center;
background-color: aquamarine;
padding: 1rem;
}
And this will give us a centered child div!
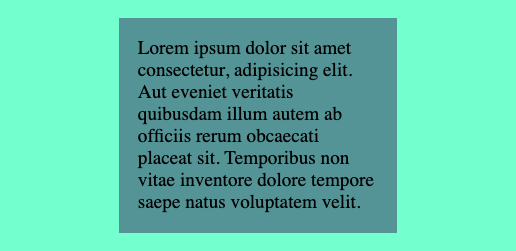
How to Center a Div Vertically?
If your parent container div
has a certain height, you can also center the child element vertically. How? By using the Flexbox align-items
property.
<div class="container">
<div class="element">
Lorem ipsum dolor sit amet consectetur, adipisicing elit. Aut eveniet veritatis quibusdam illum autem ab officiis rerum obcaecati placeat sit. Temporibus non vitae inventore dolore tempore saepe natus voluptatem velit.
</div>
</div>
.container {
display: flex;
justify-content: center;
background-color: aquamarine;
padding: 1rem;
height: 300px;
}
.element {
background-color: cadetblue;
padding: 1rem;
width: 200px;
}
The parent container has a height of 300px
. But the child element is noticeably smaller, just stretched to the full height. Like so:
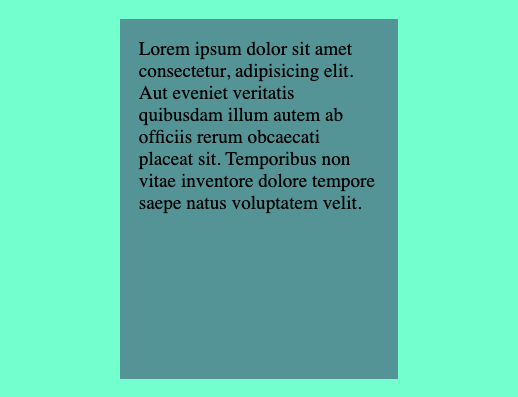
But what we want instead is that the child element is as tall as its content, and is centered both horizontally and vertically.
We achieve this by setting the align-items
property of the .container
to align-items: center;
.
.container {
display: flex;
justify-content: center;
align-items: center;
background-color: aquamarine;
padding: 1rem;
height: 300px;
}
.element {
background-color: cadetblue;
padding: 1rem;
width: 200px;
}
And this gives us a div
that is centered both horizontally and vertically! 🥳
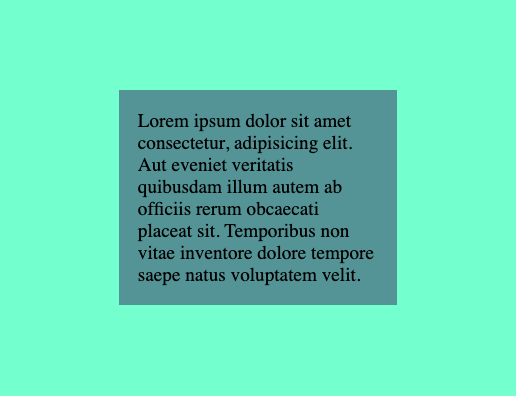
More Ways of Centering Things
There are surely some more ways of centering things with CSS. Here is a summary of the most important ones that are a bit too much to be discussed in this tutorial:
- Centering elements with
float
- Centering with CSS Grid
- Centering elements in the viewport
- Centering using Tailwind CSS
There are plenty of ways to center things in CSS. This article introduced the most common ones that you can use without any CSS framework. I hope it was helpful - feel free to come back to it whenever you need to look up how to center things! 😄